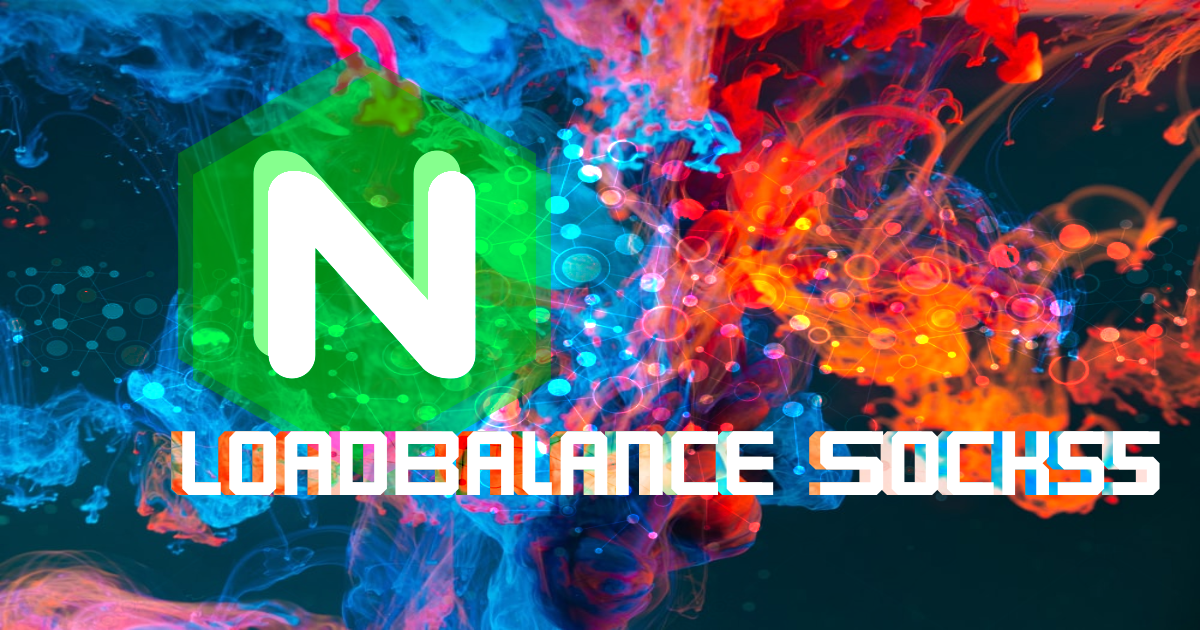
purpose
Using nginx to load balance traffic through multiple socks5 proxies.
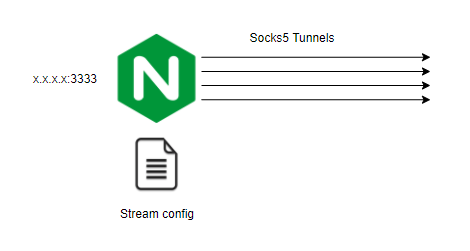
prerequisite
Nginx with the ngx_stream_core_module module .It is available since version 1.9.0.
You can use
to check if you have the correct version. Look at https://docs.nginx.com/nginx/admin-guide/installing-nginx/installing-nginx-open-source/#prebuilt_debian if you need to install the correct version.
You can check here for socks5.
configuration
By default nginx.conf is configured for http module.
So we need to change the configuration.
1 2
| mkdir /etc/nginx/stream.d
|
Edit Nginx configuration
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45
| cat nginx.conf
user nginx; worker_processes 1;
error_log /var/log/nginx/error.log warn; pid /var/run/nginx.pid;
events { worker_connections 1024; }
http { include /etc/nginx/mime.types; default_type application/octet-stream;
log_format main '$remote_addr - $remote_user [$time_local] "$request" ' '$status $body_bytes_sent "$http_referer" ' '"$http_user_agent" "$http_x_forwarded_for"';
access_log /var/log/nginx/access.log main;
sendfile on;
keepalive_timeout 65;
include /etc/nginx/conf.d/*.conf; }
stream {
log_format basic '$remote_addr:$remote_port $upstream_addr [$time_local] ' '$protocol $status $bytes_sent $bytes_received ' '$session_time';
access_log /var/log/nginx/stream-access.log basic;
include /etc/nginx/stream.d/*.conf; }
|
We add the stream{…} with some default configuration in it, like logs and stream.d folder.
Now edit a file in stream.d name it ‘default.conf’ or ‘whatever.conf’
1 2 3 4 5 6 7 8 9 10 11 12 13
| upstream stream_socks5 { server 127.0.0.1:1080; server 127.0.0.1:1081; server 127.0.0.1:1082; server 127.0.0.1:1083; }
server { listen 127.0.0.1:3333; proxy_pass stream_socks5; }
|
In stream_socks5 add the proxy socks5 you have, one by line. In this configuration I have 4 local ssh proxy socks5.
Start SSH socks5. I create a small bash script for this
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34
| #!/bin/bash
ssh_key_path='/home/kali/.ssh/superKey.pkey' user='ssh_user'
ssh_servers=$(cat <<EOF ssh.server.com:22 ssh2.someServer.com:22 x.x.x.x:2222 EOF )
port_inc=1080
for server in $ssh_servers; do
ip=$(echo $server | awk -F ":" '{print $1}') port=$(echo $server | awk -F ":" '{print $2}')
ssh -D $port_inc -C -N -f -i $ssh_key_path $user@$ip -p $port
port_inc=$(($port_inc+1))
done
pgrep -f 'ssh -D' netstat -tanlp | grep 127.0.0.1
|
Normally it start all the local port like
1 2 3
| tcp 0 0 127.0.0.1:1082 0.0.0.0:* LISTEN 7296/ssh tcp 0 0 127.0.0.1:1080 0.0.0.0:* LISTEN 7280/ssh tcp 0 0 127.0.0.1:1081 0.0.0.0:* LISTEN 7288/ssh
|
restart nginx service
1
| sudo service nginx restart
|
run multiple time
1
| curl --socks5 127.0.0.1:3333 http://myexternalip.com/raw
|
Normally the IP address change.
You can use this proxy socks5 in
- Browser
- proxychains4
- curl
- …
By default nginx use round robin to load balance stream.
You can look at https://docs.nginx.com/nginx/admin-guide/load-balancer/tcp-udp-load-balancer/ if you want to add UDP and change some configuration.